Your first task¶
How custom tasks work¶
Every task you add to Lampyre will appear in the Scripts and the List of requests windows under the category you set to the task itself.
Any task may contain not only the request execution code, but also:
When you run your task in Lampyre, it fills the corresponding tables with data. After this, you can work with this data in Lampyre, visualize it on a graph or on a map, etc.
How to create a simple task¶
Preliminary actions¶
<?xml version="1.0" encoding="utf-8"?>
<appSettings>
<add key="pythonPath" value ="C:\path\to\python.exe"/>
</appSettings>
The Lighthouse development API consists of only two files - lighthouse.py with all required classes and ontology.py with the ontology - a set of built in entities for using in your tasks.
These files can be found in the Lampyre installation directory in the user_tasks folder. You can copy them to your projects or you can create your project directories right in the user_tasks folder.
Import classes¶
To create a task you have to create a Python script first. Once it’s done, open this script and import classes from the Lighthouse library:
from lighthouse import *
Describe tables¶
Data table is the key component of any task. There should be at least one there to work with.
To describe a task’s table, the Header
metaclass is used. It describes the table name and all its columns (in API terms they are called Fields). Headers, Objects and some other entities are described in declarative style - you create them as Python classes.
The Field
class describes the column name, data type and some other optional properties.
Describe your table header by creating a class using this example:
class Products(metaclass=Header): # here you use Header as metaclass
display_name = 'Products table' # this is table name you see in UI
Name = Field('Name', ValueType.String)
Price = Field('Price', ValueType.Float)
Available = Field('Available', ValueType.Boolean)
Create a task class¶
Your custom task itself is also a Python class, which inherits the Task
class. This class contains some methods you should override in order for your task to work.
Note
if you use PyCharm, after typing your class definition you can press Ctrl + Shift + O to see all methods available for overriding
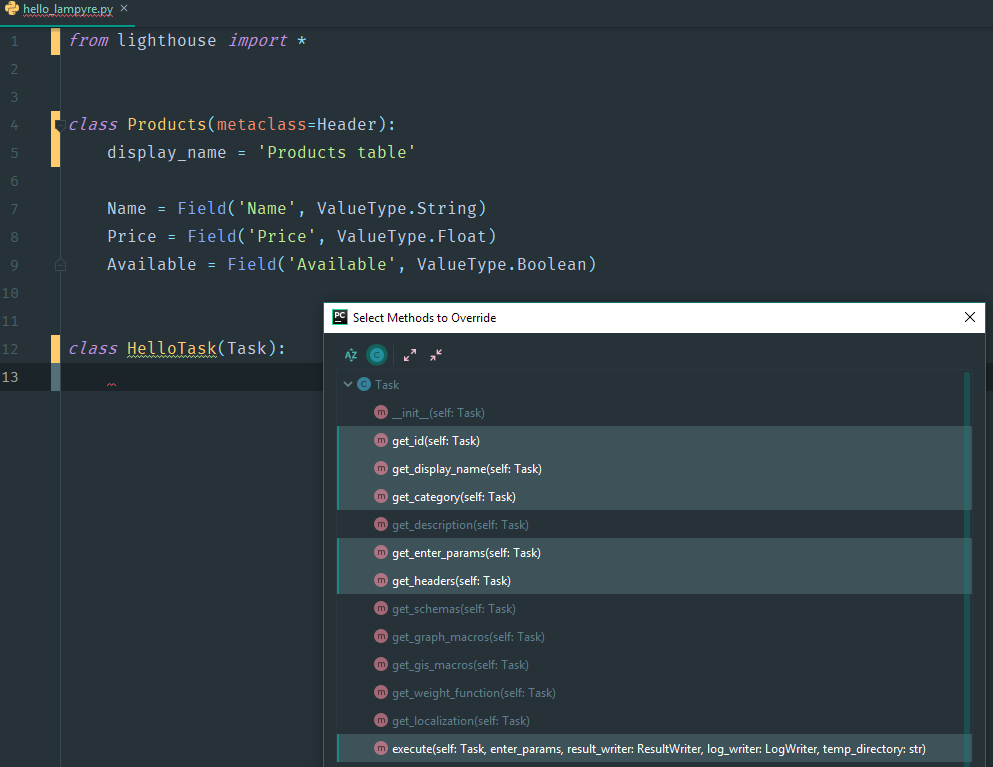
Create your task class like this:
class HelloTask(Task): # here we simply inherit Task class
# every task should have unique id string in uuid4 format
def get_id(self):
return 'b795762d-6a93-4bc6-8a42-6440d400107e'
# this is a name of category in "List of requests" dialog
def get_category(self):
return 'Tutorial tasks'
# this is your task name
def get_display_name(self):
return 'Hello Lampyre task'
# here you return task table(s) headers
def get_headers(self):
return ProductsHeader
# this is input for your task. In this example we don't need it,
# so we return an empty collection
def get_enter_params(self):
return EnterParamCollection()
# here goes task execution payload (see next paragraph)
def execute(self, enter_params, result_writer, log_writer, temp_directory):
pass
Write execution code¶
In simple terms, task execution is filling the task tables with data. All the execution code goes to the execute()
method of your task class.
To put data in a table (to create rows) the ResultWriter
class is used. An instance of this class is passed to the execute()
method by Lampyre.
Table rows have the structure of a Python dictionary, where keys are header fields and values are the data for the corresponding cells. Such row, once passed to the write_line()
method, will be added to the table.
Alter your execute()
code os it loks like this:
def execute(self, enter_params, result_writer, log_writer, temp_directory):
result_writer.write_line({
Products.Name: "Avocado",
Products.Price: 1.95,
Products.Available: True
})
The code above will add one row to a table. Once the execute()
method is exited, the task will finish its work and Lampyre will receive the result data.
You can now upload your task script to Lampyre
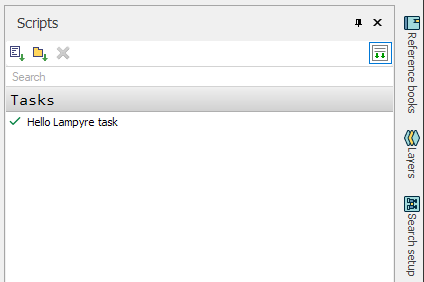
Select it in the List of requests and click Execute
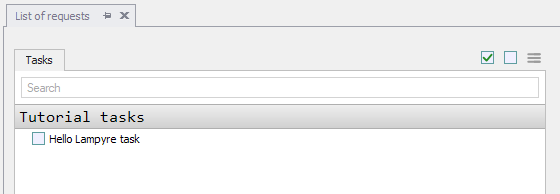
Open your table in the Requests window:
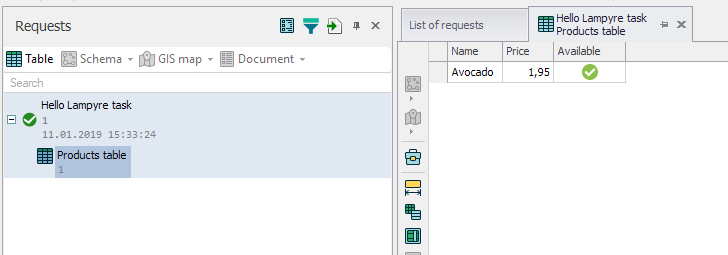
Congratulations! Your first task is ready!