Ontology¶
What is ontology¶
Ontology is a collection of entities (attributes, objects, links) and schemas, shared by multiple tasks and gathered in one place. Structurally it is a Python script that contains code for such things.
System ontology¶
System ontology, that you will get with Lampyre out of the box, contains all of the attributes, objects and links, that are available in Lampyre itself. That means, that if you want to use such system entities as IP, Domain, Facebook account, and so on, in the schemas for your own tasks, you don’t have to describe them in code - you can just import them from the ontology.
System ontology is located in the ontology.py file.
Note
If you use any ontology, the system one or your own, you should upload it to Lampyre alongside your scripts.
from lighthouse import *
from ontology import IP, Domain, IPToDomain
class ExampleHeader(metaclass=Header):
display_name = 'System entities'
IP = Field('IP', ValueType.String)
Domain = Field('Domain', ValueType.String)
Date = Field('Date', ValueType.Datetime)
class ExampleSchema(metaclass=Schema):
name = 'Example schema'
Header = ExampleHeader
ip = SchemaObject(type=IP, mapping={IP.IP: Header.IP})
domain = SchemaObject(type=Domain, mapping={Domain.Domain: Header.Domain})
ip_to_domain = SchemaLink(type=IPToDomain, mapping={IPToDomain.Resolved: Header.Date}, begin=ip, end=domain)
After filling the table with data, you can create a schema with built-in objects:
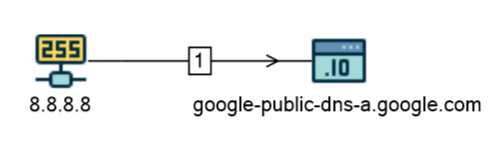
In your type definitions you can also use attributes from the system collection, called Attributes
. This collection properties are the attributes of the system entities:
Attributes also have shortcuts for creating new attribute types - they are methods, named after the types, like Attributes.str(), Attributes.float(), etc.
Custom ontologies¶
You can create your own custom ontologies to store your types and share them among many tasks.
You can also store your headers, schemas, and everything you want in the ontology script, as shareable functions.
Every ontology must have a unique ID, which can be set by creating a variable ONTOLOGY_ID with a uuid4 format string. You can also set a display name for your ontology, which you will see in the Lampyre UI.
from lighthouse import Attribute, Object, Link, Header, Schema
ONTOLOGY_ID = 'efd0ef64-cb95-47cc-a1c8-98b166548a11'
NAME = 'My ontology'