Task enterparams¶
What are enterparams?¶
Enterparameters, or just parameters, represent forms of input data for your requests - each of them have a type, a name and other optional properties. When you fill them in the List of requests dialog or use the macro feature (on a graph or a GIS map), Lampyre sends them to your task’s execute()
method.
Parameters in the code¶
Describing task parameters¶
Task parameter is described by an instance of the EnterParamField
class. When you upload your task to Lampyre, Lampyre calls for the task’s get_enter_params
method to get all possible parameters.
So, in order to create parameters one has to override the get_enter_params
method. This method returns the EnterParamCollection
instance:
def get_enter_params(self):
return EnterParamCollection(
EnterParamField('file_path', 'File', ValueType.String, file_path=True, required=True, category='Required'),
EnterParamField('urls', 'URLs', ValueType.String, is_array=True, value_sources=[Attributes.System.URL],
category='Optional'),
EnterParamField('timeout', 'Timeout', ValueType.Integer, default_value=60, category='Optional'),
EnterParamField('presets', 'Presets', ValueType.String, predefined_values=['Quick', 'Brown', 'Fox'],
category='Required', description='Jumps over the lazy dog')
)
This is how these parameters look like in the Lampyre UI:
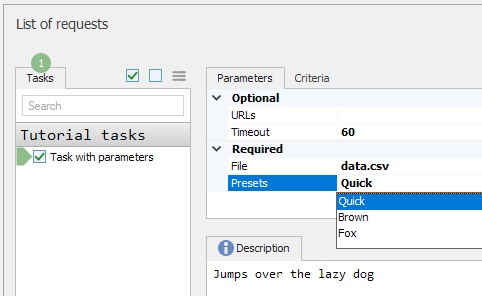
You can also use the add()
and add_enter_param()
methods to fill in this collection. Parameters in the collection my be accessed by their system_name in the dict
style (as keys).
EnterParamField class¶
The EnterParamField
class has a large set of constructor options (required parameters are starred):
system_name*
- This is a unique parameter internal name used in theexecute()
method
display_name*
- This is a parameter name for the UI
type*
- Just like theField
, all parameters have one of theValueType
data types
is_array
- This flag indicates that the parameter may have multiple values (only for string parameters)
required
- Tasks cannot be run until this parameter has some value
default_value
- Default value for this parameter
predefined_values
- List of preset valid values, that can’t be changed (Dropdown menu)
file_path
- Parameter is a path to file (Open file dialog)
geo_json
- Indicates that parameter is GeoJson string
value_sources
- List of attributes that can be a source for parameter value (see Schema)
category
- UI group for parameter (General by default)
description
- Short description for parameter
Using parameters at runtime¶
When Lampyre starts your task, it passes all parameters to the execute()
first argument, enter_params, as a namedtuple instance. The parameter values are stored in attributes, accessible by parameter’s system_name.
For example, this parameter from the above example
EnterParamField('file_path', 'File', ValueType.String, file_path=True, required=True, category='Required')
can be accessed in execute()
by a file_path attribute:
def execute(self, enter_params, result_writer, log_writer, temp_directory):
with open(enter_params.file_path) as fp:
...
Value sources¶
Concept¶
Manual input is not the only way to fill in task parameters before sending a request in Lampyre. You can also select entities on a graph or a GIS map, or select an area on a map, and do the following:
Right-click and select To requests
Right-click and run a macro
First option will open the filtered List of requests dialog with filled in parameters, second will immediately run a task with these parameters and automatically enrich your graph or map with new entities (This is what Macro does).
How does this work? Every parameter can have an instruction to receive value(s) from one or more attributes (Both objects and links can have attributes). These instructions are passed to the EnterParamField
constructor using the value_sources parameter
Setting value sources for parameters¶
The ValueSource
class is used to set a source of parameter’s values. You can pass Attribute
or an attribute name to the constructor.
class Site(metaclass=Object):
URL = Attribute('URL', ValueType.String)
...
# some parameter for task, accepting URL attribute as value source
EnterParamField('urls', 'URLs', ValueType.String, value_sources=[ValueSource(Site.URL)])
...
Another capability of ValueSource
is changing other parameters when activated (but not on manual input). Constructor parameters param_switch and value_switch are used for this.
For example, let’s say you want to increase the timeout parameter to value 120 when urls are filled by ValueSource
. You can script this behavior the following way:
# param_switch is a system_name of another parameter
EnterParamField('urls', 'URLs', ValueType.String,
value_sources=[ValueSource(Site.URL, param_switch='timeout', value_switch=120)])
Here is this value source in action:
Icons for custom objects provided by Icons 8